Mastering Inheritance in C++: A Comprehensive Guide
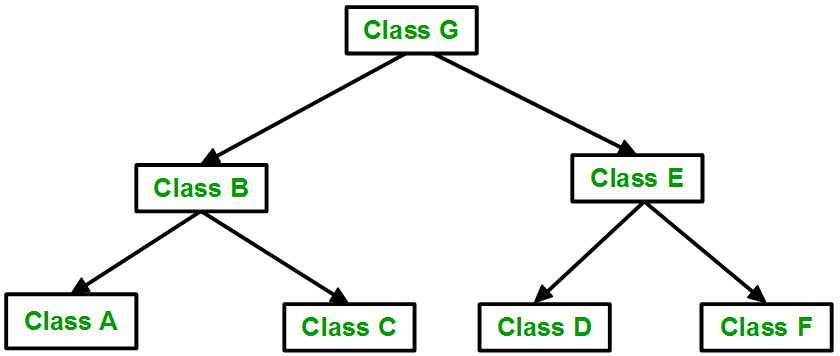
Introduction: Inheritance is a powerful feature of object-oriented programming (OOP) that allows you to create new classes (derived classes) based on existing classes (base classes). It enables code reuse, promotes modularity, and facilitates the creation of hierarchical relationships between classes. In C++, inheritance is a core concept that forms the foundation of polymorphism, encapsulation, and abstraction. Understanding how to implement inheritance in C++ is essential for building complex and maintainable software systems. In this comprehensive guide, we’ll explore the fundamentals of inheritance in C++, covering everything from basic syntax to advanced techniques. By the end of this article, you’ll have a deep understanding of how to leverage inheritance to create flexible and extensible C++ programs.
- Introduction to Inheritance: Inheritance is a mechanism in C++ that allows a class (derived class) to inherit properties and behaviors from another class (base class). The derived class automatically gains access to the members (variables and functions) of the base class, allowing for code reuse and specialization. Inheritance enables the creation of class hierarchies, where classes are organized in a tree-like structure based on their relationships.
- Basic Syntax of Inheritance: In C++, inheritance is implemented using the
class
keyword followed by a colon:
and the access specifier (public
,protected
, orprivate
) followed by the name of the base class. Here’s the basic syntax of inheritance:
class BaseClass {
// Base class members
};class DerivedClass : access-specifier BaseClass {
// Derived class members
};
The access-specifier
determines the visibility of the inherited members in the derived class. By default, if no access specifier is provided, the members are inherited as private
.
- Types of Inheritance: C++ supports several types of inheritance, including:
- Single Inheritance: Where a derived class inherits from a single base class.
- Multiple Inheritance: Where a derived class inherits from multiple base classes.
- Multilevel Inheritance: Where a derived class serves as the base class for another derived class, forming a chain of inheritance.
- Hierarchical Inheritance: Where multiple derived classes inherit from a single base class.
- Hybrid Inheritance: Combination of multiple inheritance types.
Each type of inheritance has its own advantages and use cases, and it’s important to choose the appropriate type based on the requirements of your application.
- Access Specifiers in Inheritance: In C++, access specifiers (
public
,protected
, andprivate
) play a crucial role in determining the visibility of inherited members in the derived class:
public
: Inherited members are accessible to everyone.protected
: Inherited members are accessible to the derived class and its subclasses.private
: Inherited members are not accessible in the derived class.
Here’s an example demonstrating the use of access specifiers in inheritance:
class Base {
public:
int publicVar;
protected:
int protectedVar;
private:
int privateVar;
};class Derived : public Base {
// publicVar is accessible
// protectedVar is accessible
// privateVar is not accessible
};
- Constructors and Destructors in Inheritance: In C++, constructors and destructors are not inherited by derived classes. However, the constructor of the base class is automatically called when an object of the derived class is created. You can explicitly call the base class constructor using the initializer list syntax in the derived class constructor. Here’s an example:
class Base {
public:
Base() {
cout << "Base class constructor" << endl;
}
~Base() {
cout << "Base class destructor" << endl;
}
};class Derived : public Base {
public:
Derived() : Base() {
cout << "Derived class constructor" << endl;
}
~Derived() {
cout << "Derived class destructor" << endl;
}
};
In this example, the base class constructor is called before the derived class constructor.
- Function Overriding: Function overriding is a key aspect of inheritance that allows a derived class to provide its own implementation of a function inherited from the base class. To override a function, the function signature and return type in the derived class must match exactly with the function in the base class. Here’s an example of function overriding:
class Base {
public:
void display() {
cout << "Base class display function" << endl;
}
};class Derived : public Base {
public:
void display() {
cout << "Derived class display function" << endl;
}
};
In this example, the display()
function in the derived class overrides the display()
function in the base class.
- Virtual Functions and Polymorphism: Virtual functions are functions declared in the base class with the
virtual
keyword, allowing them to be overridden in derived classes. Virtual functions enable runtime polymorphism, where the appropriate function to call is determined at runtime based on the object’s actual type. To declare a virtual function, use thevirtual
keyword in the base class and override it in the derived class. Here’s an example:
class Base {
public:
virtual void display() {
cout << "Base class display function" << endl;
}
};class Derived : public Base {
public:
void display() override {
cout << "Derived class display function" << endl;
}
};
In this example, the display()
function in the derived class overrides the virtual function in the base class.
- Abstract Classes and Pure Virtual Functions: An abstract class is a class that cannot be instantiated and contains one or more pure virtual functions. A pure virtual function is a virtual function that is declared in the base class but has no implementation. Abstract classes serve as interfaces for derived classes, defining a common interface for a group of related classes. To declare a pure virtual function, use the
virtual
keyword followed by= 0
. Here’s an example:
class Shape {
public:
virtual void draw() = 0; // Pure virtual function
};class Circle : public Shape {
public:
void draw() override {
cout << "Drawing a circle" << endl;
}
};
class Rectangle : public Shape {
public:
void draw() override {
cout << "Drawing a rectangle" << endl;
}
};
In this example, the Shape
class is an abstract class with a pure virtual function draw()
. The Circle
and Rectangle
classes inherit from the Shape
class and provide their own implementations of the draw()
function.
- Accessing Base Class Members: In C++, derived classes have access to inherited members of the base class based on the access specifiers. You can access base class members using the scope resolution operator
::
. Here’s an example:
class Base {
public:
int baseVar;
};class Derived : public Base {
public:
void display() {
cout << "Base variable: " << baseVar << endl;
}
};
In this example, the display()
function in the derived class accesses the baseVar
member of the base class.
- Conclusion: Congratulations! You’ve completed this comprehensive guide on how to implement inheritance in C++. Inheritance is a powerful feature of C++ that allows for code reuse, specialization, and hierarchical relationships between classes. By mastering inheritance, you gain the ability to create flexible and extensible C++ programs that are easier to maintain and scale. Keep experimenting, exploring, and incorporating inheritance into your C++ projects to unlock its full potential. Happy coding!