Mastering String Manipulation in Swift: A Comprehensive Guide
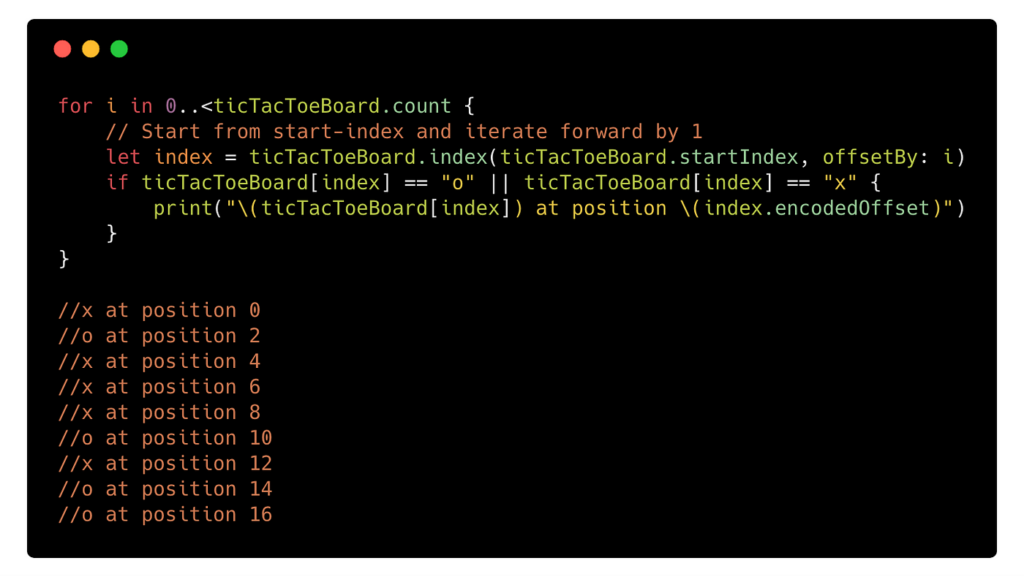
Introduction: Strings are fundamental data types in Swift, representing sequences of characters. Swift provides a rich set of features and functions for manipulating strings, enabling developers to perform a wide range of operations such as concatenation, substring extraction, searching, and formatting. Whether you’re building iOS, macOS, watchOS, or tvOS applications, understanding how to manipulate strings in Swift is essential for implementing user interfaces, handling user input, and processing text data. In this comprehensive guide, we’ll explore the various techniques and best practices for string manipulation in Swift, covering everything from basic operations to advanced functionalities. By the end of this article, you’ll be equipped with the knowledge and skills to handle strings effectively and efficiently in your Swift projects.
- Creating Strings: In Swift, strings can be created using string literals, which are sequences of characters enclosed in double quotes
" "
. Here’s an example:
let greeting = "Hello, World!"
You can also create strings from other data types using string interpolation, which allows you to embed expressions within string literals. Here’s an example:
let name = "John"
let message = "Hello, \(name)!"
- String Concatenation: String concatenation is the process of combining two or more strings into a single string. In Swift, you can concatenate strings using the
+
operator or the+=
compound assignment operator. Here’s an example:
let firstName = "John"
let lastName = "Doe"
let fullName = firstName + " " + lastName
Alternatively, you can use the +=
operator to append a string to an existing string:
var greeting = "Hello"
greeting += ", World!"
- String Interpolation: String interpolation allows you to include expressions, variables, and constants within string literals to create dynamic strings. In Swift, string interpolation is performed using the backslash
\
followed by parentheses()
. Here’s an example:
let name = "John"
let age = 30
let message = "Hello, my name is \(name) and I am \(age) years old."
In this example, the values of the name
and age
variables are interpolated into the string message
.
- String Length: You can determine the length of a string in Swift using the
count
property or thecount
method of theString
type. Here’s an example:
let text = "Hello, World!"
let length = text.count
print("Length of string: \(length)")
- Substrings: A substring is a portion of a string, typically extracted based on a range of indices. In Swift, you can extract substrings using subscript notation or the
prefix(_:)
,suffix(_:)
, anddropFirst(_:)
,dropLast(_:)
methods. Here are examples of extracting substrings:
let text = "Hello, World!"
let substring1 = text[..<text.index(text.startIndex, offsetBy: 5)]
let substring2 = text.suffix(6)
let substring3 = text.dropFirst(7)
let substring4 = text.dropLast(7)
In these examples, substring1
extracts the first 5 characters, substring2
extracts the last 6 characters, substring3
removes the first 7 characters, and substring4
removes the last 7 characters.
- String Searching: Swift provides several methods for searching within strings, such as
contains(_:)
,hasPrefix(_:)
, andhasSuffix(_:)
. These methods allow you to check if a string contains a specific substring, starts with a certain prefix, or ends with a particular suffix. Here are examples of string searching:
let text = "Hello, World!"
let containsHello = text.contains("Hello")
let startsWithHello = text.hasPrefix("Hello")
let endsWithWorld = text.hasSuffix("World!")
In these examples, containsHello
is true
, startsWithHello
is true
, and endsWithWorld
is true
.
- String Case Conversion: Swift provides methods for converting the case of strings, such as
uppercased()
andlowercased()
. These methods allow you to convert a string to uppercase or lowercase characters. Here are examples of string case conversion:
let text = "Hello, World!"
let uppercaseText = text.uppercased()
let lowercaseText = text.lowercased()
In these examples, uppercaseText
contains "HELLO, WORLD!"
and lowercaseText
contains "hello, world!"
.
- String Splitting and Joining: You can split a string into substrings based on a delimiter using the
split(separator:)
method. Conversely, you can join an array of strings into a single string using thejoined(separator:)
method. Here are examples of string splitting and joining:
let text = "apple,banana,orange"
let fruits = text.split(separator: ",")
let joinedText = fruits.joined(separator: ";")
In these examples, fruits
is an array containing "apple"
, "banana"
, and "orange"
, and joinedText
contains "apple;banana;orange"
.
- String Formatting: String formatting allows you to create formatted strings with placeholders for dynamic values. Swift provides several formatting options, including string interpolation, the
String(format:)
initializer, and theNSLocalizedString(_:tableName:bundle:value:comment:)
function for localization. Here are examples of string formatting:
let name = "John"
let age = 30
let formattedString1 = "Hello, my name is \(name) and I am \(age) years old."
let formattedString2 = String(format: "Hello, my name is %@ and I am %d years old.", name, age)
let localizedString = NSLocalizedString("Hello", tableName: "Greetings", bundle: .main, value: "", comment: "")
In these examples, formattedString1
and formattedString2
produce the same output using different formatting methods, and localizedString
retrieves a localized string from a specified table.
- Conclusion: Congratulations! You’ve completed this comprehensive guide on how to manipulate strings in Swift. String manipulation is a fundamental aspect of Swift programming, enabling you to process text data, create dynamic user interfaces, and implement various algorithms and logic in your Swift applications. By mastering string manipulation techniques and understanding the rich set of functionalities provided by Swift’s string API, you gain the ability to handle strings effectively and efficiently in your projects. Keep experimenting, exploring, and incorporating string manipulation into your Swift development workflow to unlock its full potential. Happy coding!