Mastering Exception Handling in Python: A Comprehensive Guide
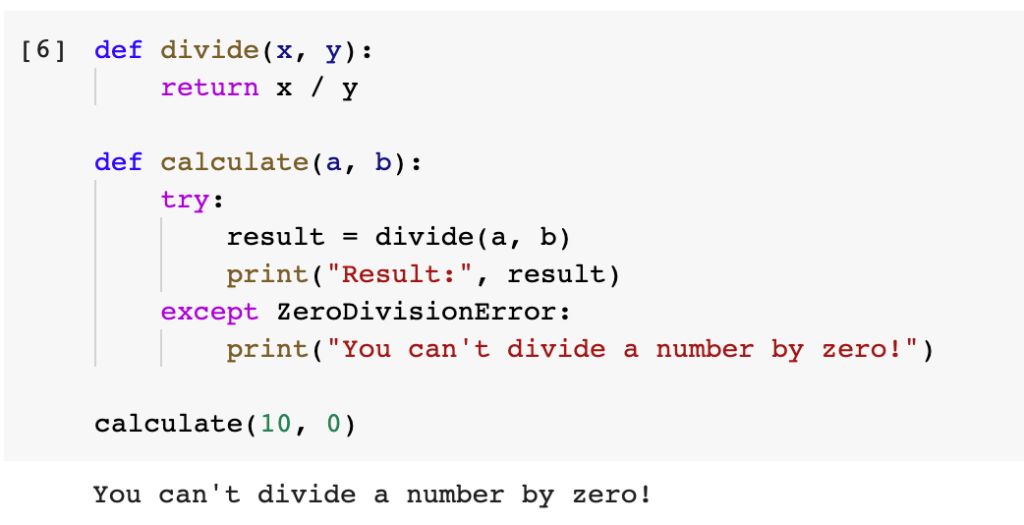
Introduction: Exception handling is a critical aspect of writing robust and reliable Python code. It allows developers to gracefully handle errors, unexpected situations, and exceptional conditions that may arise during program execution. Python’s exception handling mechanism provides a structured way to detect, report, and recover from errors, ensuring that programs remain stable and responsive. In this comprehensive guide, we’ll explore the fundamentals of exception handling in Python, covering everything from basic syntax to advanced techniques. By the end of this article, you’ll be equipped with the knowledge and skills to handle exceptions effectively and write resilient Python code.
- Understanding Exceptions in Python: In Python, an exception is an error that occurs during the execution of a program, disrupting the normal flow of control. Exceptions can be caused by various factors, such as invalid input, file I/O errors, arithmetic errors, and runtime conditions. When an exception occurs, Python raises an exception object, which contains information about the error type, message, and traceback. By handling exceptions, you can intercept and respond to errors gracefully, preventing program crashes and data corruption.
- Basic Syntax of Exception Handling: Python provides a try-except block for handling exceptions. The syntax is as follows:
try:
# Code block where an exception may occur
except ExceptionType:
# Code block to handle the exception
Inside the try
block, you place the code that may raise an exception. If an exception occurs, Python jumps to the corresponding except
block and executes the code inside it. You can specify the type of exception to catch after the except
keyword, or use a generic except
block to catch any exception.
- Handling Specific Exceptions: You can handle specific types of exceptions by specifying the exception class after the
except
keyword. This allows you to customize the handling logic based on the type of error encountered. Here’s an example:
try:
num = 10 / 0
except ZeroDivisionError:
print("Error: Division by zero")
In this example, the ZeroDivisionError
exception is caught and the corresponding error message is printed.
- Handling Multiple Exceptions: You can handle multiple types of exceptions using multiple
except
blocks or a singleexcept
block with multiple exception types separated by commas. Here’s an example:
try:
value = int(input("Enter a number: "))
result = 10 / value
except ValueError:
print("Error: Invalid input. Please enter a valid number.")
except ZeroDivisionError:
print("Error: Division by zero")
In this example, both ValueError
and ZeroDivisionError
exceptions are handled separately.
- The
else
andfinally
Blocks: Python allows you to includeelse
andfinally
blocks in exception handling code for additional control flow:
- The
else
block is executed if no exceptions occur in thetry
block. - The
finally
block is always executed, regardless of whether an exception occurs or not. It’s typically used for cleanup operations, such as closing files or releasing resources.
Here’s an example:
try:
file = open("example.txt", "r")
except FileNotFoundError:
print("Error: File not found")
else:
print("File contents:")
print(file.read())
file.close()
finally:
print("Cleanup: Closing file")
In this example, the else
block prints the contents of the file if it exists, and the finally
block closes the file regardless of whether an exception occurs.
- Raising Exceptions: You can raise exceptions manually using the
raise
statement. This allows you to signal errors or exceptional conditions explicitly within your code. Here’s an example:
def calculate_age(year):
if year < 0:
raise ValueError("Invalid year. Year must be a positive number.")
return 2024 - yeartry:
age = calculate_age(-1990)
print("Age:", age)
except ValueError as e:
print("Error:", e)
In this example, the calculate_age
function raises a ValueError
if the input year is negative.
- Custom Exception Classes: Python allows you to define custom exception classes by subclassing the built-in
Exception
class or one of its subclasses. Custom exception classes can provide additional context and information about specific types of errors in your application. Here’s an example:
class CustomError(Exception):
passdef process_data(data):
if not data:
raise CustomError("No data found. Please provide valid data.")
try:
process_data([])
except CustomError as e:
print("Error:", e)
In this example, the CustomError
exception is raised when no data is provided to the process_data
function.
- Exception Propagation: In Python, exceptions propagate up the call stack until they are caught by an exception handler. If an exception is not caught, it results in the termination of the program and the display of a traceback. You can use the
except
statement without specifying an exception type to catch all exceptions and prevent them from propagating further. - Best Practices for Exception Handling: When handling exceptions in Python, consider the following best practices:
- Be specific: Catch specific types of exceptions whenever possible to handle errors appropriately.
- Use try-except sparingly: Only catch exceptions that you know how to handle, and let other exceptions propagate up the call stack.
- Provide informative error messages: Include relevant information in error messages to aid in debugging and troubleshooting.
- Use
finally
for cleanup: Use thefinally
block to ensure that cleanup operations are always performed, even if an exception occurs.
- Conclusion: Congratulations! You’ve completed this comprehensive guide on how to handle exceptions in Python. Exception handling is a crucial aspect of writing robust and reliable Python code, allowing you to detect, report, and recover from errors gracefully. By mastering exception handling techniques and best practices, you can ensure that your Python programs remain stable, resilient, and maintainable. Keep experimenting, exploring, and refining your exception handling skills to become a proficient Python developer. Happy coding!